In Microsoft Dynamics 365 development, managing data flow between functions and services is crucial, yet challenging. Developers often grapple with the need to maintain strongly typed data while ensuring flexibility for future extensions. This blog post explores the role of data contracts in addressing this issue. We’ll delve into when, why and how to use data contracts to enhance your development process, reducing technical debt and future-proofing your solutions. Through practical examples, we’ll demonstrate how data contracts can simplify your code, making it more robust and adaptable to evolving business needs.
What Is a Data Contract?
A data contract is a formal agreement that defines how data is exchanged between different components within a system, ensuring consistency and clarity in communication. In Microsoft Dynamics 365 development, data contracts are particularly valuable when passing data between functions and services. They help maintain strongly typed data, which minimizes errors and enhances the robustness of your code. The primary benefit of using data contracts is that they lay the foundation for future extensions without the need for significant rework. By encapsulating data in a defined structure, developers can introduce new variables or business logic without altering the core method signatures, thus reducing technical debt and promoting long-term scalability.
Data contracts differ significantly from Service Level Agreements (SLAs). While an SLA is an agreement between service providers and clients outlining the expected level of service, a data contract is an internal agreement within the codebase, ensuring that data passed between components adheres to a specific structure. Unlike SLAs, which focus on service outcomes and performance metrics, data contracts focus on the precise structure and type of data being exchanged, making them a key tool in maintaining code quality and enabling efficient debugging.
By using data contracts in your Dynamics 365 development, you ensure that your solutions are not only meeting current business needs but are also well-positioned to adapt to future requirements.
A common problem we encounter in Microsoft Dynamics 365 development is how to pass data between functions and services. In this post we will review strategies that balance the needs of strongly typed data without introducing technical debt. We’ll explore how we can write our solution today which supports extension tomorrow.
Data Contract Structure
The structure of a data contract in Dynamics 365 is built upon key components that ensure data is exchanged in a consistent and strongly typed manner. This is achieved through the use of attributes like DataContractAttribute
and DataMemberAttribute
, which are applied to classes and their members to define how data should be serialized and deserialized.
At the core, a data contract is a class that encapsulates the data you wish to exchange. The class itself is decorated with the DataContractAttribute
, signaling to the system that this class is intended to be used as a data contract. Each member of the class that you want to include in the data exchange is decorated with the DataMemberAttribute
, which makes it part of the data contract.
Who Uses Data Contracts?
Data contracts play a crucial role in various aspects of software development, particularly in environments that involve complex data exchanges. Understanding who uses data contracts can help determine if the content applies to you or if you should be considering the details in your own work.
- Software Developers: Developers working with Dynamics 365, X++, or similar platforms often use data contracts to define the structure and types of data being passed between functions and services. By using data contracts, developers can ensure data integrity, simplify debugging and facilitate future extensions of their code.
- System Architects: System architects responsible for designing and overseeing the architecture of applications and services leverage data contracts to establish clear data exchange protocols. This ensures that different system components interact seamlessly and that data is consistently managed across the system.
- Integration Specialists: Professionals involved in integrating different systems or services use data contracts to define the data exchanged between disparate systems. This helps in creating robust integration points that are easy to manage and maintain.
- Business Analysts: Business analysts may use data contracts to understand the data requirements and flows within an application. This understanding helps in defining requirements more accurately and ensures that the data contracts align with business needs.
- Quality Assurance Engineers: QA engineers testing applications with complex data exchanges use data contracts to validate the correctness and consistency of data. They ensure that data contracts are properly implemented and that data flows as expected between different system components.
Understanding the role of data contracts is essential for these professionals as it helps in creating well-structured, maintainable, and scalable solutions. If you find yourself in one of these roles, or if you are involved in any aspect of software development that deals with data management, considering the details of data contracts is crucial for the success of your projects.
Writing a Data Contract: Example and Overview
Creating and using data contracts effectively involves several key steps:
How to Create a New Class
Begin by defining a new class for the data contract, using DataContractAttribute
to mark it as a data contract.
Implementing the Necessary Interfaces
The class should implement DataContractAttribute
, and its properties should be marked with DataMemberAttribute
to ensure proper serialization and deserialization.
How to Define Properties
Properties within the data contract should be defined with DataMemberAttribute
and include getter and setter methods for data access and modification.
Best Practices for Naming Conventions and Attribute Use
- Naming Conventions: Use clear, descriptive names for properties.
- Attribute Use: Apply
DataContractAttribute
to the class andDataMemberAttribute
to properties. - Error Handling/Validation: Implement data validation and error handling to maintain data integrity.
Serialization and Deserialization Process
Data contracts are serialized into XML or JSON format and deserialized back into objects, ensuring consistent data formatting.
Security Factors to Protect Data
Implement encryption and follow secure coding practices to protect sensitive data within your contracts.
How to Test Contracts
Conduct unit and integration tests to ensure data contracts are correctly serialized, deserialized and integrated with other components.
Integration with D365 F&O Operations
Ensure data contracts are correctly used in Dynamics 365 F&O operations, such as service operations and batch jobs, for smooth data exchanges.
Let us examine a method that asks for a lot of data and returns very little. Imagine a business requirement to process quantity updates for sales lines. We could write our business logic, exposing individual arguments.
public boolean processQtyUpdate(InventTransId _inventTransId,
InventQty _origQty,
InventQty _newQty,
boolean _showError = false)
{
boolean ok = true;
//Do work that solves today's problem
return ok;
}
This approach enforces exchange of strongly typed data but limits our ability to extend this function to meet future business needs. Since chain of command/event subscriptions cannot change the method signature, individually defined arguments are set in stone until the next platform update.
Making a Request
We should approach the problem by submitting a request contract instead of individual arguments.
public boolean processQtyUpdateBetter(SalesLineQtyUpdateRequestContract _request)
{
boolean ok = true;
SalesLine salesLine = SalesLine::findInventTransId(_request.InventTransId());
//Do work that solves today's problem and enables new requirements
return ok;
}
This approach, coupled with an extensible pattern of hydrating the request contract, allows extensions to add new data input to our processor method. The method signature does not need to change to support future business requirements. The input data is strongly typed and easy to read in the debugger.
Writing a Data Contract
Data contacts are easy to create. They are classes with protected (by default) global variables exposed through public get/set (parm) methods. When using data contracts with SysOp or reporting, the class should be decorated with DataContractAttribute, and each parm method should be decorated with DataMemberAttribute.
[DataContract()]
class SalesLineQtyUpdateRequestContract
{
InventTransId inventTransId;
[DataMember()]
public InventTransId InventTransId(InventTransId _inventTransId = inventTransId)
{
inventTransId = _inventTransId;
return inventTransId;
}
}
Returning a Response
Data contracts are also our preferred approach to return multiple pieces of data to the caller (instead of a container of data).
public SalesLineQtyUpdateResponseContract
processQtyUpdateUltimate(SalesLineQtyUpdateRequestContract _request)
{
SalesLineQtyUpdateResponseContract response = new SalesLineQtyUpdateResponseContract();
SalesLine salesLine = SalesLine::findInventTransId(_request.InventTransId());
try
{
//Do work that solves today's problem and enables new requirements
}
catch
{
response.Fault(true);
}
return response;
}
Arguments Against Containers
One could argue accepting and returning a container would achieve the same ends. Though true, we discourage this anti-pattern. The container does not ensure strongly typed contents. The extension story for containers requires unknown parties to play well with each other and requires unnecessary amounts of data validation when extracting the data from the container index. Containers are harder to read when debugging. Utilizing a container to pass our request and response data will only shift technical debt from the “cannot extend” to “where’s my notes on how to parse this return.”
In this blog, we have shown that by utilizing data contracts, the groundwork is laid to meet future business requirements via extension. Additionally, contracts allow handshake agreement on variables between callers and support developer productivity by being easier to read and extend.
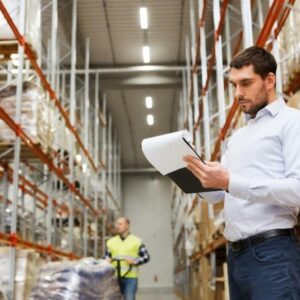
Hidden Treasures of Advanced Warehousing
Make the most of Microsoft Dynamics 365’s Advanced Warehousing module and gain insight into the hidden treasures to unlock value.
Tools and Resources
When working with data contracts in Dynamics 365, leveraging available tools and resources can significantly aid in overcoming challenges and enhancing your understanding. Here are some valuable resources to consider:
- Using Data Contracts in X++: A comprehensive guide on implementing and using data contracts in X++, providing essential information for Dynamics AX developers.
- Data Contracts in Cloud Scale Analytics: This resource covers the role of data contracts in cloud-scale analytics, offering insights into how they fit into broader cloud architectures.
- Microsoft Dynamics Community Forum – Data Contracts: A forum where users discuss issues and share solutions related to data contracts in Dynamics 365. It’s a great place to ask questions and find community-driven answers.
- Upgrading AX 2012 to D365: enVista’s Approach: While focused on upgrades, this blog provides valuable insights into how data contracts play a role in the transition from AX 2012 to Dynamics 365.
- Microsoft Dynamics Community Forum – Data Contracts Thread: Another helpful forum thread discussing data contracts, offering practical advice and solutions from the Dynamics community.
These resources provide a wealth of information and support for navigating data contracts in Dynamics 365, helping you tackle challenges and improve your development practices.
Deep Expertise in Dynamics AX and D365 Development
enVista is dedicated to helping the Microsoft community by continuously sharing our in-depth knowledge of X++ and Microsoft Dynamics 365 development. If you are struggling with Dynamics AX or D365 development, contact us, and we will connect you with one of our in-house experts.
Read our other topics in our D365 Developer Series: